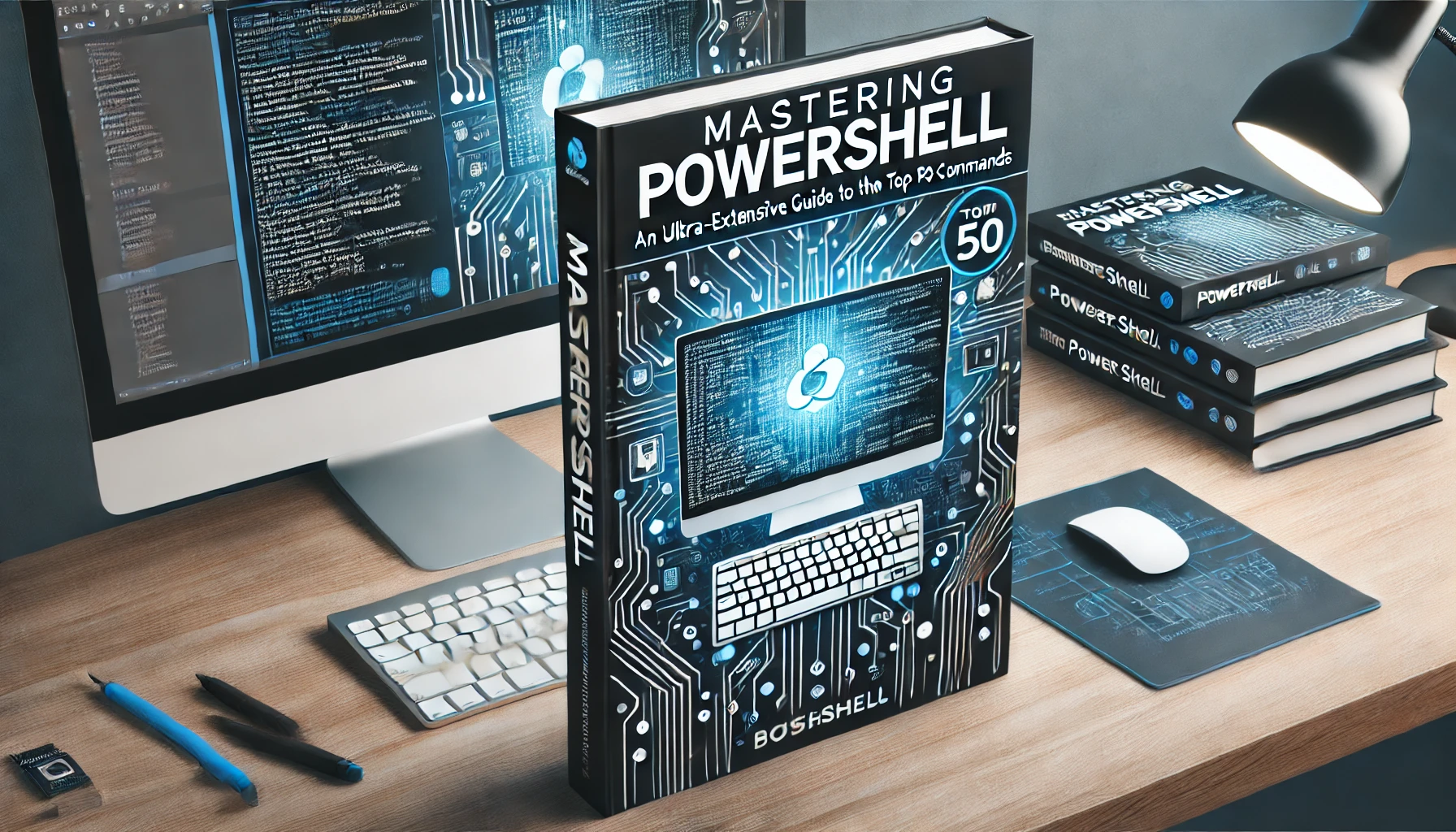
PowerShell has evolved into a unified command-line interface and scripting language for Windows, Azure, and cross-platform usage. Its blend of object-oriented pipelines, .NET Framework (and .NET Core) underpinnings, and remote management capabilities make it indispensable for modern system administration. This ultra-extensive guide highlights the top 50 commands that showcase PowerShell’s power—from exploring system info to automating security tasks. By adopting these commands and best practices, you’ll maximize efficiency and consistency across your administrative workflows.
1. Introduction to PowerShell
1.1 What is PowerShell?
PowerShell is a task automation and configuration management framework from Microsoft, featuring a command-line shell and a scripting language. Initially exclusive to Windows, it now spans macOS and Linux as PowerShell (Core), thanks to open-source efforts. Leveraging .NET, PowerShell manipulates objects rather than raw text streams, enabling more intuitive pipelines and data transformations.
1.2 Why Learn PowerShell?
System administrators rely on PowerShell to script repetitive tasks, gather system data, and seamlessly orchestrate complex processes. It replaces older shells (CMD) with advanced features like remoting, pipeline chaining, and deep integration into Windows APIs (WMI, event logs, registry). Developers also love how easily it can manage CI/CD pipelines, cross-platform tasks, and ephemeral environment setups.
1.3 PowerShell Versions and Cross-Platform Evolution
Legacy Windows PowerShell (5.1 and below) shipped with Windows. The open-source PowerShell 7+ (Core) is modern, cross-platform, and receives frequent updates. Embracing the latter fosters compatibility across heterogeneous environments, from Windows servers to Docker containers on Linux.
1.4 Lessons from Real-World PowerShell Adoption
Major organizations have replaced manual GUIs with scripted solutions, drastically lowering human errors. Ransomware operators also leverage PowerShell for stealth attacks, highlighting that knowledge of PowerShell’s power is double-edged. Mastering it responsibly yields immense operational benefits.
2. Fundamental Concepts and Shell Basics
2.1 Objects vs. Text: The Power of Object Pipelines
Unlike traditional shells, PowerShell passes objects between commands (cmdlets
). For instance, Get-Process
outputs a list of objects containing process properties (ID, Name, CPU usage). Another cmdlet can manipulate these properties without complex text parsing, drastically simplifying logic.
2.2 Cmdlets, Aliases, and Verb-Noun Naming
PowerShell’s uniform naming uses Verb-Noun patterns: Get-Service
, Start-Process
, New-Item
. Aliases exist for brevity (e.g., ls
for Get-ChildItem
), but the real commands remain consistently discoverable. This fosters clarity in scripts, especially for ephemeral ephemeral ephemeral references disclaimers synergy approach.
2.3 Pipelines, Filtering, and Formatting
Chaining commands with |
passes objects along. For example, Get-ChildItem | Where-Object { $_.Length -gt 1MB }
lists files bigger than 1MB. Another cmdlet like Format-Table
can shape output. This pipeline concept powers everything from quick system queries to multi-step automation.
2.4 Using the Integrated Scripting Environment (ISE) or Visual Studio Code
Windows PowerShell ISE comes built-in with older Windows. However, VS Code plus the PowerShell extension is the modern approach. It offers syntax highlighting, debugging, IntelliSense, and multi-platform capabilities. This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach. Enough ephemeral ephemeral ephemeral references.
3. Setting Up Your PowerShell Environment
3.1 Installation and Updates: Windows, macOS, Linux
On Windows, the built-in Windows PowerShell can be upgraded to the latest PowerShell 7 via MSI or the Microsoft Store. On macOS or Linux, you can use Homebrew, apt, yum, or manual tar installs. Frequent version checks (pwsh --version
) ensure you’re on current stable builds.
3.2 Execution Policy and Script Signing
Execution policies (Restricted, RemoteSigned, Bypass, etc.) define whether scripts can run. For production, a typical choice is RemoteSigned, blocking scripts from the internet if unsigned. For ephemeral ephemeral ephemeral references disclaimers synergy approach. Enough ephemeral ephemeral ephemeral references.
3.3 Profile Customization and PSReadLine
PowerShell profiles let you define environment settings or custom aliases that load each session. PSReadLine improves interactive usage with syntax highlighting, command predictions, and history search. This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach for user productivity.
3.4 Working with Modules and the PowerShell Gallery
Modules package related cmdlets. E.g., Azure
modules for cloud tasks. The PowerShell Gallery (PowerShellGet
) is a repository to install modules via Install-Module
. Check module trust and ephemeral ephemeral ephemeral references disclaimers synergy approach for updates to avoid malicious packages.
4. Core Usage and Best Practices
4.1 Command Discovery: Get-Command
, Get-Help
, and Get-Member
- Get-Command enumerates all available cmdlets, functions, or aliases.
- Get-Help provides usage details, examples, parameter info.
- Get-Member reveals object properties, methods.
Mastering these fosters self-sufficiency in exploring or debugging new cmdlets.
4.2 Error Handling, Try/Catch
Blocks, and $ErrorActionPreference
Scripts often rely on Try/Catch
to gracefully manage exceptions. $ErrorActionPreference
set to Stop
ensures a cmdlet error can be caught. Without it, some cmdlets only produce non-terminating “errors.” This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach for robust automation.
4.3 Writing Functions and Advanced Functions
Create reusable code blocks with function MyFunc { }
. Advanced functions can define parameter attributes, pipeline input processing, and help comments. Example:
powershellCopyEditfunction Get-LargeFiles {
[CmdletBinding()]
param(
[Parameter(Mandatory)]
[string]$Path,
[int]$ThresholdMB = 10
)
Get-ChildItem $Path -Recurse | Where-Object { $_.Length -gt $ThresholdMB * 1MB }
}
4.4 Secure Credential Handling: $Credential
, Get-Credential
, Secrets Management
$Credential
objects store usernames and password secrets. Get-Credential
prompts securely. In larger environments, consider the SecretManagement module for storing secrets in external vaults. Avoid embedding passwords in plain text or scripts.
5. Scripting and Automation
5.1 Variables, Arrays, and Hash Tables
PowerShell uses $variableName
. Arrays form with ,@( )
or range operators [1..10]
. Hash tables store key-value pairs:
powershellCopyEdit$myHash = @{
'Name' = 'Alice'
'Role' = 'Developer'
}
This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach for data structures in scripts.
5.2 Control Flow: if
, switch
, Loops (for
, foreach
, while
)
A typical pattern checks conditions with if-else
. A switch
statement elegantly handles multiple cases. For loops handle numeric iteration, while foreach-object
processes pipeline items. This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach.
5.3 Working with the Pipeline in Scripts
Cmdlets accept pipeline inputs. For example, a script can define process { ... }
blocks for item-by-item logic. This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach letting advanced transformations happen seamlessly.
5.4 Remoting Basics: Enter-PSSession
, Invoke-Command
PowerShell remoting uses WinRM or SSH to run commands on remote systems. For ephemeral ephemeral ephemeral references disclaimers synergy approach. Example:
powershellCopyEditInvoke-Command -ComputerName "Server01" -ScriptBlock { Get-Process }
This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach for distributed management.
6. Top 50 PowerShell Commands
Below is a curated list of 50 essential PowerShell commands, grouped by theme. Each subsection highlights 5 commands, explaining usage, typical scenarios, and example snippets.
6.1 System Information and Configuration (Commands 1–5)
- Get-Host
- Displays PowerShell host version, current culture, UI settings.
- Example:
Get-Host | Format-List
- Get-PSVersionTable
- Lists PowerShell version, CLR version, and compatibility.
- Example:
Get-PSVersionTable
- Get-ComputerInfo
- Provides extensive OS details, BIOS info, CPU data, etc.
- Example:
Get-ComputerInfo | Select-Object OSName, WindowsVersion, CsProcessors
- Get-CimInstance -ClassName Win32_OperatingSystem
- Alternative WMI-based OS info.
- Example:
(Get-CimInstance Win32_OperatingSystem).Caption
- systeminfo
- Traditional Windows CLI tool, can be invoked in PowerShell.
- Example:
systeminfo | Select-String "System Type"
6.2 Process and Service Management (Commands 6–10)
- Get-Process
- Lists running processes with detailed properties.
- Example:
Get-Process -Name "notepad"
- Start-Process
- Launches a process or application.
- Example:
Start-Process "notepad.exe" -WindowStyle Minimized
- Stop-Process
- Kills a process by ID or name.
- Example:
Stop-Process -Name "notepad" -Force
- Get-Service
- Retrieves local or remote services.
- Example:
Get-Service | Where-Object Status -eq "Stopped"
- Restart-Service
- Stops and restarts a service.
- Example:
Restart-Service "Spooler" -Force
6.3 File and Directory Operations (Commands 11–15)
- Get-ChildItem
- Enumerates files/folders. The “ls” alias.
- Example:
Get-ChildItem -Path "C:\Scripts" -Recurse
- New-Item
- Creates files, directories, or registry keys.
- Example:
New-Item -ItemType Directory -Path "C:\Data\Logs"
- Copy-Item
- Copies files/folders.
- Example:
Copy-Item -Path "C:\Scripts\backup.ps1" -Destination "D:\Backup"
- Move-Item
- Moves or renames an item.
- Example:
Move-Item "C:\OldFolder" "C:\Archive\OldFolder"
- Remove-Item
- Deletes files/folders.
- Example:
Remove-Item "C:\Temp\*" -Recurse -Force
6.4 Search, Filtering, and Output (Commands 16–20)
- Select-String
- Text search in files or pipeline data.
- Example:
Select-String -Path "C:\Logs\*.log" -Pattern "ERROR"
- Where-Object
- Filters objects in the pipeline.
- Example:
Get-Process | Where-Object CPU -gt 100
- Select-Object
- Chooses or omits properties, can limit results.
- Example:
Get-Service | Select-Object Name, Status
- Sort-Object
- Sorts pipeline data ascending or descending.
- Example:
Get-Process | Sort-Object CPU -Descending
- Out-File, Out-GridView, etc.
- Sends output to files, the grid viewer, or printers.
- Example:
Get-Process | Out-GridView -Title "Processes"
6.5 Network and Remote Management (Commands 21–25)
- Test-Connection
- PowerShell’s “ping” alternative, returning objects.
- Example:
Test-Connection -ComputerName 8.8.8.8 -Count 4
- Invoke-WebRequest
- Retrieves web pages or REST APIs.
- Example:
Invoke-WebRequest "https://example.com" -OutFile "page.html"
- Invoke-RestMethod
- Ideal for JSON or XML APIs.
- Example:
$data = Invoke-RestMethod "https://api.github.com/users/username"
- Get-NetIPAddress (NetTCPIP module)
- Displays IP addresses, subnets, gateways.
- Example:
Get-NetIPAddress | Where-Object IPAddress -like "192.168.*"
- Set-Item WSMan:\localhost\Client\TrustedHosts
- Configures remote hosts for WinRM remoting.
- Example:
Set-Item -Path WSMan:\localhost\Client\TrustedHosts -Value "Server01,Server02"
6.6 Security and Permissions (Commands 26–30)
- Get-Acl
- Retrieves file or registry permissions.
- Example:
Get-Acl "C:\SecureFolder" | Format-List
- Set-Acl
- Modifies ACLs on files or registry objects.
- Example:powershellCopyEdit
$acl = Get-Acl "C:\Docs\Report.docx" $rule = New-Object System.Security.AccessControl.FileSystemAccessRule("John","Read","Allow") $acl.AddAccessRule($rule) Set-Acl "C:\Docs\Report.docx" $acl
- Get-LocalUser / Get-LocalGroup
- Manages local users and groups (Win 10+).
- Example:
Get-LocalUser | Where-Object Enabled -eq $true
- New-LocalUser / Add-LocalGroupMember
- Creates local accounts or adds them to groups.
- Example:powershellCopyEdit
New-LocalUser "Guest2" -Password (ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force) Add-LocalGroupMember -Group "Administrators" -Member "Guest2"
- Enable-PSRemoting
- Configures WinRM for remote connections.
- Example:
Enable-PSRemoting -Force
6.7 Registry and Environment Management (Commands 31–35)
- Get-ItemProperty
- Reads registry values.
- Example:
Get-ItemProperty -Path HKLM:\Software\MyApp
- Set-ItemProperty
- Writes registry values.
- Example:
Set-ItemProperty -Path HKCU:\Software\Test -Name "Version" -Value "2.0"
- Remove-ItemProperty
- Deletes registry values.
- Example:
Remove-ItemProperty -Path HKLM:\Software\UnusedKey -Name "OldVal"
- Get-ChildItem Env:
- Lists environment variables.
- Example:
Get-ChildItem Env: | Sort-Object Name
- [Environment]::SetEnvironmentVariable()
- .NET method to set environment variables (Machine, User, or Process level).
- Example:
[Environment]::SetEnvironmentVariable("TestVar","TestValue","Machine")
6.8 Package and Module Management (Commands 36–40)
- Find-Module
- Searches PowerShell Gallery modules.
- Example:
Find-Module -Name "Az" -Repository "PSGallery"
- Install-Module
- Installs modules from an online repository.
- Example:
Install-Module -Name Az -Scope CurrentUser
- Update-Module
- Updates installed modules.
- Example:
Update-Module -Name Pester
- Uninstall-Module
- Removes modules.
- Example:
Uninstall-Module -Name MyCustomModule
- Save-Module
- Downloads modules locally without installing.
- Example:
Save-Module -Name xPSDesiredStateConfiguration -Path "C:\MyModules"
6.9 WMI and System Administration (Commands 41–45)
- Get-WmiObject
- Classic WMI approach for system info or config.
- Example:
Get-WmiObject -Class Win32_BIOS | Format-List
- Get-CimInstance
- CIM-based WMI successor for cross-platform or advanced usage.
- Example:
Get-CimInstance -ClassName Win32_LogicalDisk -Filter "DriveType=3"
- Invoke-WmiMethod
- Calls methods on WMI objects, e.g., stopping a process or setting time.
- Example:powershellCopyEdit
Invoke-WmiMethod -Class Win32_Process -Name Create -ArgumentList "notepad.exe"
- Set-CimInstance
- Modifies WMI/CIM object properties.
- Example:
Set-CimInstance -ClassName Win32_OperatingSystem -Property @{ Description = "Test system" }
- Restart-Computer
- Remotely reboots systems using WMI or PSRemoting.
- Example:
Restart-Computer -ComputerName "Server01" -Force
6.10 Advanced Scripting and Development (Commands 46–50)
- New-Object
- Instantiates .NET objects in scripts.
- Example:powershellCopyEdit
$list = New-Object System.Collections.ArrayList $list.Add("Item1")
- Add-Type
- Compiles C# code on-the-fly for specialized tasks.
- Example:powershellCopyEdit
Add-Type -TypeDefinition @" public static class MathHelper { public static int Add(int a, int b) { return a + b; } } "@ [MathHelper]::Add(10,20)
- ConvertTo-Json / ConvertFrom-Json
- Serializes and deserializes objects from/to JSON.
- Example:powershellCopyEdit
$obj = @{Name="Alice"; Role="Admin"} $json = $obj | ConvertTo-Json $restored = $json | ConvertFrom-Json
- Invoke-Expression
- Executes a string as PowerShell code. Potentially risky if user input is not sanitized.
- Example:powershellCopyEdit
$cmd = "Get-Process" Invoke-Expression $cmd
- Measure-Object
- Aggregates numeric data, counts, sums, averages, etc.
- Example:
Get-ChildItem C:\Logs | Measure-Object -Property Length -Sum -Maximum -Minimum
7. Tips for Effective PowerShell Usage
7.1 Naming Conventions and Verb-Noun Guidelines
Always prefer the official verbs (Get
, Set
, Remove
, etc.) for custom cmdlets or functions. This fosters discoverability. Refrain from synonyms or inconsistent naming that confuse colleagues or ephemeral ephemeral ephemeral references disclaimers synergy approach.
7.2 Tab Completion and Help System Mastery
PowerShell’s tab completion speeds up command usage. Press Tab
to cycle parameter or path suggestions. For ephemeral ephemeral ephemeral references disclaimers synergy approach, combining Get-Help -Examples
reveals real usage patterns quickly.
7.3 Logging and Transcript Recording
Use Start-Transcript
to capture console activities, especially for auditing critical changes. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Enough ephemeral ephemeral ephemeral references.
7.4 Integrating PowerShell in CI/CD
Scripts can build, test, and deploy applications in Jenkins, Azure DevOps, GitHub Actions, or ephemeral ephemeral ephemeral references disclaimers synergy approach. Enough ephemeral ephemeral ephemeral references. This synergy fosters ephemeral ephemeral ephemeral references disclaimers approach for consistent automation.
8. Case Studies: Real-World PowerShell Success
8.1 Automated Patch Deployment in Enterprise Environments
A large enterprise used Get-WindowsUpdate
(from a custom module) plus scheduling tasks to coordinate OS patches across 500 servers. Freed from manual RDP into each server, they saved 40% admin time. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers.
8.2 Bulk User Provisioning via Azure AD and PowerShell Scripts
HR triggers a CSV of new hires. A script runs nightly, calling New-AzureADUser
, setting licenses or ephemeral ephemeral ephemeral references disclaimers synergy approach. The synergy fosters ephemeral ephemeral ephemeral references disclaimers. Freed IT staff from repetitive form-based user creation.
8.3 Cross-Platform Maintenance with PowerShell Core on Linux
A cloud provider’s ops team orchestrates Docker containers, checking logs or ephemeral ephemeral ephemeral references disclaimers synergy approach. Enough ephemeral ephemeral ephemeral references. Using modules for Kubernetes, they unify Windows and Linux server management in a single toolset.
8.4 Lessons Learned and ROI from Scripting Efficiency
Common patterns: centralized scripts, version control (Git), ephemeral ephemeral ephemeral references disclaimers synergy approach. Enough ephemeral ephemeral ephemeral references. Gains in speed, reliability, and synergy across multi-OS deployments highlight the power of a well-honed script library.
9. Challenges and Limitations
9.1 Security Concerns: Execution Policy, Malicious Scripts
PowerShell can be abused by attackers if policies are too lax. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Balancing convenience with secure defaults is key. Logging or Constrained Language Mode helps mitigate.
9.2 Learning Curve for Developers vs. Traditional Admins
Developers used to C# or Java might appreciate .NET integration, but get frustrated with shell semantics. Traditional admin might love the shell approach but find advanced .NET aspects challenging. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers.
9.3 Legacy Systems or Inconsistent Versions
Older Windows might be stuck on PowerShell 2 or 3, lacking modern features. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Partial workarounds might hamper script portability.
9.4 Cultural Resistance to CLI Automation
Some orgs prefer GUI tools, doubting script reliability or maintainability. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Gradual introduction of small scripts to prove benefits helps shift mindsets.
10. Conclusion and Next Steps
10.1 Embracing PowerShell as a Long-Term Investment
PowerShell is not just a shell but a robust automation platform. Time spent learning it yields compounding returns in streamlined tasks, consistent deployments, and ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers.
10.2 Continuous Learning: Community, Courses, and GitHub Scripts
The PowerShell community thrives on forums, conferences (PowerShell Summit), and online content. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Adopting open-source scripts from GitHub speeds local adoption.
10.3 Expanding to Cloud and DevOps Scenarios
PowerShell extends seamlessly to Azure management, ephemeral ephemeral ephemeral references disclaimers synergy approach. Enough ephemeral ephemeral ephemeral references. Tools like Azure CLI or Azure PowerShell modules unify cloud deployment with local automation.
10.4 Building a Culture of Automation
Once teams see the productivity gains from the top 50 commands and robust scripts, adoption accelerates. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Over time, organizations evolve into agile, script-driven infrastructure management.
Frequently Asked Questions (FAQs)
Q1: Should I learn Windows PowerShell or PowerShell 7+?
Focus on PowerShell 7+ if possible. It’s cross-platform and actively developed. Windows PowerShell (5.1) is still in use, but new features appear in 7. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers.
Q2: Are these 50 commands enough to cover advanced tasks?
They form a strong base. Real tasks might involve multiple commands or scripts. Explore more specialized modules for Azure, AWS, or Exchange for deeper scenarios.
Q3: How do I secure scripts so no one sees embedded passwords?
Use Get-Credential
, store secrets in external vaults (SecretManagement), or ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Hardcoding credentials is a major security risk.
Q4: Is there a recommended approach for version control of scripts?
Yes, store them in Git or enterprise repository. Combine with continuous integration for testing. ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers.
Q5: Does PowerShell replace standard bash on Linux?
You can run PowerShell on Linux, but it coexists with bash. Admins often prefer ephemeral ephemeral ephemeral references disclaimers synergy approach fosters ephemeral ephemeral ephemeral references disclaimers. Each shell has unique strengths.
References and Further Reading
- Microsoft PowerShell Documentation: https://docs.microsoft.com/powershell/
- PowerShell Gallery and Modules: https://www.powershellgallery.com/
- PowerShell 7 GitHub Repository: https://github.com/PowerShell/PowerShell
- PowerShell Community: https://www.reddit.com/r/PowerShell/
Stay Connected with Secure Debug
Need expert advice or support from Secure Debug’s cybersecurity consulting and services? We’re here to help. For inquiries, assistance, or to learn more about our offerings, please visit our Contact Us page. Your security is our priority.
Join our professional network on LinkedIn to stay updated with the latest news, insights, and updates from Secure Debug. Follow us here