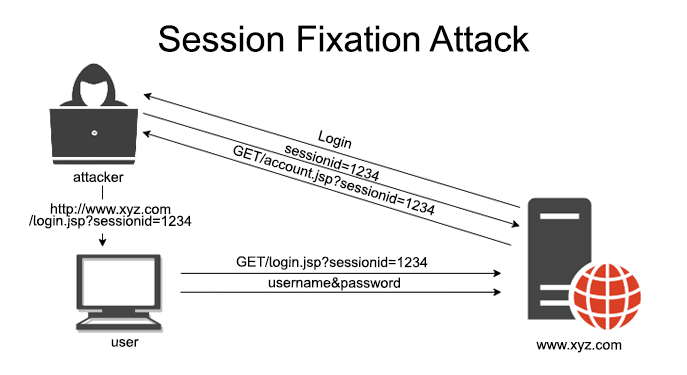
Introduction:
Session Fixation is a type of web application vulnerability that allows an attacker to hijack a user’s session by obtaining their session identifier. This blog post will dive into the details of Session Fixation, provide real-world examples, explore effective detection techniques, and offer robust prevention strategies with code samples.
Understanding Session Fixation:
Session Fixation occurs when an attacker is able to fix a user’s session identifier (such as a cookie or a URL parameter) before the user logs in, and then use that identifier to impersonate the user after they have successfully authenticated. This vulnerability arises when a web application fails to properly manage user sessions and does not assign new session identifiers upon authentication.
Case Study: Cookie-Based Session Fixation
Consider a web application that uses cookies to store user session identifiers. If the application does not issue a new session identifier upon successful login, an attacker could send a victim a link containing a predetermined session identifier, trick the victim into logging in, and then hijack their session using the predetermined identifier.
Detecting Session Fixation Vulnerabilities:
Detecting Session Fixation vulnerabilities typically involves manual testing and the use of proxy tools such as Burp Suite. By observing the application’s session management behavior and manipulating session identifiers, security testers can identify potential vulnerabilities.
Preventing Session Fixation Vulnerabilities:
- Session Regeneration: Assign a new session identifier upon successful user authentication to ensure that any previously fixed session identifiers become invalid.
Example using Python and Flask:
from flask import Flask, session, redirect, url_for
from flask_login import LoginManager, login_user
app = Flask(__name__)
login_manager = LoginManager(app)
@app.route('/login', methods=['POST'])
def login():
username = request.form.get('username')
password = request.form.get('password')
user = authenticate(username, password)
if user:
session.regenerate() # Regenerate session identifier
login_user(user)
return redirect(url_for('dashboard'))
else:
return 'Invalid credentials'
- Secure Session Cookies: Set the Secure and HttpOnly flags on session cookies to ensure they are transmitted only over HTTPS and are not accessible through JavaScript.
- Use Built-In Session Management: Many web application frameworks provide built-in session management features that include secure practices to help prevent Session Fixation attacks. Utilize these features whenever possible.
- Validate Session Identifiers: Ensure that session identifiers are only accepted from trusted sources, such as server-generated cookies, and not from user-supplied input like URL parameters or POST data.
Conclusion:
Understanding, detecting, and mitigating Session Fixation vulnerabilities is crucial for maintaining strong web application security. By implementing the preventative measures and incorporating code samples outlined in this blog post, you can significantly reduce the risk of unauthorized access and protect your users’ sensitive data.